Best Practices for Secure Coding in PowerShell: A Guide
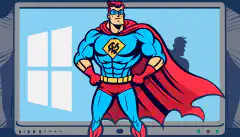
Table of Contents
PowerShell is a popular task automation and configuration management framework that is used to automate repetitive tasks and simplify management of Windows-based systems. As with any programming language, PowerShell code can be vulnerable to security threats if developers do not follow secure coding standards. In this article, we will discuss best practices for secure coding in PowerShell.
Input Validation
User input is often a significant source of security risks. Input validation is the process of verifying that the user input meets the expected criteria and is safe to use in the application.
For example, when a user enters a password, the input should meet the password policy of the application. To validate the input, developers can use built-in functions such as Test-Path
or regular expressions to ensure that the input meets the expected criteria.
function Validate-Input {
param(
[string]$input
)
if ($input -match "^[A-Za-z0-9]+$") {
return $true
}
else {
return $false
}
}
Avoid Using Unsafe Functions
PowerShell has several functions that can be vulnerable to security issues if not used carefully. Functions such as Invoke-Expression, Get-Content, and ConvertTo-SecureString can allow attackers to execute malicious code. Developers should avoid using these functions or use them with caution by restricting input parameters and using them only when necessary.
For example, instead of using Invoke-Expression function to execute a command, developers should use Start-Process.
# Instead of using Invoke-Expression
Invoke-Expression "Get-ChildItem"
# Use Start-Process
Start-Process "Get-ChildItem"
Use Cryptography Libraries
Cryptography libraries such as .NET Cryptography and Bouncy Castle provide a secure way to perform encryption and decryption operations. Use these libraries instead of creating custom encryption methods, which may be prone to vulnerabilities.
For example, to encrypt a password, use the .NET Cryptography library as follows:
$securePassword = ConvertTo-SecureString "MyPassword" -AsPlainText -Force
$encryptedPassword = [System.Convert]::ToBase64String($securePassword.ToByteArray())
Follow the Principle of Least Privilege
The principle of least privilege is a security best practice that restricts users or processes to the minimum level of access necessary to perform their functions. This means that users should only have access to the resources they need to do their job and nothing more.
Developers should follow this principle when writing code to minimize the impact of security breaches. By limiting the access of a program or user, the risk of a successful attack is reduced.
For example, if an application requires read-only access to a database, it should use a database account with read-only permissions instead of an account with full permissions. This reduces the risk of an attacker exploiting the application to modify or delete data. Similarly, if a user only needs to perform certain tasks, they should not be given administrator-level access to the system.
By following the principle of least privilege, developers can reduce the potential damage of a security breach and limit the scope of the attack.
Keep Libraries and Frameworks Updated
Libraries and frameworks can contain security vulnerabilities that can be exploited by attackers. Developers should keep their libraries and frameworks updated to the latest version to avoid potential security issues.
When a security vulnerability is discovered in a library or framework, the developers of that library or framework will usually release a patch or update to fix the vulnerability. It’s important to keep up to date with these updates and apply them as soon as possible to minimize the risk of a security breach.
For example, if the application uses a third-party library, such as Pester
, which has had a security vulnerability, the developer should update to the latest version of the library that addresses the vulnerability. This ensures that the application is not susceptible to attacks that exploit the vulnerability.
By keeping libraries and frameworks updated, developers can ensure that their code is more secure and less vulnerable to attacks. It’s important to make sure that all dependencies are up to date and that any known security vulnerabilities have been patched.
Use a Static Code Analyzer
A static code analyzer is a tool that can identify potential security vulnerabilities in the code before it is executed. By analyzing the code before deployment, developers can detect and fix security issues before they become a problem.
In PowerShell, there are several static code analyzers available, such as PSScriptAnalyzer
. This tool can detect issues such as hard-coded passwords, improper use of environment variables, and use of unsafe functions.
For example, PSScriptAnalyzer
is a popular static code analyzer that examines PowerShell code for potential security vulnerabilities. It can detect issues such as:
- Hard-coded credentials
- Use of deprecated or unsafe functions
- Insufficient input validation
- Improper use of variables and loops
By using a static code analyzer, developers can identify and fix security vulnerabilities early in the development process. This can help to prevent security breaches and minimize the impact of any successful attacks.
Use Secure Coding Practices for PowerShell Scripts
PowerShell scripts are vulnerable to several security risks such as code injection, privilege escalation, and data leakage. To ensure the security of PowerShell scripts, developers should follow secure coding practices such as:
Sanitize Input and Output
Sanitizing user input is important to prevent code injection attacks. Developers should validate and sanitize user input to ensure that it meets expected criteria and does not contain malicious code. Additionally, when writing output to a log file or other destination, developers should sanitize any sensitive data before writing it to the file to prevent data leakage.
Use Secure Communication Protocols
When transmitting data over the network, use secure communication protocols such as HTTPS, SSL/TLS, or SSH. These protocols encrypt the data in transit, making it more difficult for attackers to intercept and manipulate the data. Conversely, avoid using unencrypted protocols such as HTTP or Telnet, as they can be easily intercepted and manipulated by attackers.
Implement Logging and Monitoring
Implement logging and monitoring mechanisms to detect and respond to security incidents in a timely manner. By logging all relevant events and setting up alerts to notify administrators of suspicious activity, developers can quickly identify and respond to security incidents before they become major issues.
Harden the Environment
Harden the environment by applying security policies and configurations to the operating system, network devices, and applications. This helps to reduce the attack surface and prevent unauthorized access. For example, developers and administrators can:
- Disable unnecessary services and protocols to reduce the attack surface
- Enforce strong passwords and password policies to prevent unauthorized access
- Configure firewalls and intrusion detection systems to prevent and detect attacks
- Implement software updates and patches to fix known security vulnerabilities
Perform Regular Vulnerability Scans
Perform regular vulnerability scans on the systems and applications to identify and remediate security vulnerabilities. Use tools such as Nessus, OpenVAS, or Microsoft Baseline Security Analyzer (MBSA) to perform these scans. Regular vulnerability scans can help to identify vulnerabilities and weaknesses in the environment, allowing developers to remediate them before they are exploited by attackers.
Educate Users and Administrators
Educate users and administrators on secure coding practices and the risks associated with insecure code. Provide training and resources to help them understand how to write secure code and how to identify and respond to security incidents. By educating users and administrators, developers can build a culture of security and promote good security practices throughout the organization.
By following these best practices, developers can ensure that their PowerShell code is secure and resilient to security threats. They can reduce the risk of successful attacks and minimize the impact of any security incidents that do occur.
Conclusion
PowerShell is a powerful tool for automating tasks and managing Windows-based systems, but it’s important to follow secure coding practices to avoid security vulnerabilities. In this article, we’ve covered best practices for secure coding in PowerShell, including input validation, avoiding unsafe functions, using cryptography libraries, and more.
By implementing these practices, developers can reduce the risk of security breaches and protect their systems and data. It’s important to stay up to date with the latest security threats and vulnerabilities, and to continuously improve the security posture of PowerShell code. With the right tools and practices, PowerShell can be a secure and reliable tool for managing and automating systems.
References
- PowerShell documentation
- Top 25 Series - Software Errors
- Input Validation Cheat Sheet
- Guide to General Server Security