Unsafe and Insecure C# Functions: Secure Coding Practices and Alternatives for Stronger Software
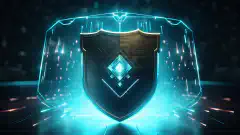
Table of Contents
Unsafe C# Functions and Their Secure Alternatives
In the world of programming, C# stands as a potent and widely-adopted language lauded for its versatility and robustness. Yet, like any programming language, it harbors functions that, when used carelessly, can expose vulnerabilities. In this comprehensive discourse, we embark on an exploration of unsafe C# functions that wield the potential to bestow security perils upon applications, and concurrently, we delve into their secure alternatives. By apprehending these pitfalls and mastering the art of mitigation, developers can forge code that epitomizes security and dependability.
Introduction
In the realm of application development, C# has emerged as a favored companion, facilitating the construction of an array of software, encompassing both desktop applications and web solutions. While this programming language furnishes developers with an array of functions and capabilities, it also harbors certain functions that can, unwittingly, become conduits for ushering in security vulnerabilities. In the ensuing sections, we venture into an in-depth analysis of these functions, unearthing their associated security quandaries and charting a course to navigate these treacherous waters.
The Perils of Unsafe C# Functions
Within the realm of C# programming, certain functions loom as veritable pitfalls, offering adversaries avenues to exploit vulnerabilities such as buffer overflows, injection attacks, and data leaks. It is paramount for developers to embrace a heightened awareness of these lurking dangers and conscientiously opt for fortified alternatives to forestall potential exploits.
String Manipulation
Unsafe: Using string.Format()
The utilization of string.Format()
introduces a precarious avenue for format string vulnerabilities, a fertile ground for potential exploits. In this scenario, attackers could cunningly inject malicious format specifiers, thus unfurling a realm of unauthorized data disclosure and in some dire instances, ushering in the nefarious specter of remote code execution.
// Using string.Format()
string name = "John";
int age = 30;
string formattedString = string.Format("My name is {0} and I am {1} years old.", name, age);
Console.WriteLine(formattedString);
Secure Alternative: Leveraging string interpolation
To fortify the code’s resilience against the lurking perils of format string vulnerabilities, a more judicious approach lies in the embrace of string interpolation. By adopting this practice, developers unfurl a twofold benefit: not only does it bestow heightened code readability, but it also meticulously executes string formatting. As a result, the specter of format string vulnerabilities is quelled, ensuring a robust bastion against potential exploits.
// Using string interpolation
string name = "John";
int age = 30;
string interpolatedString = $"My name is {name} and I am {age} years old.";
Console.WriteLine(interpolatedString);
Input Validation
Unsafe: Insufficient Input Validation
Underestimating the gravity of comprehensive user input validation opens the floodgates to a spectrum of vulnerabilities, including the notorious SQL injection and the omnipresent threat of cross-site scripting (XSS) attacks.
string userInput = Request.QueryString["input"];
string sqlQuery = "SELECT * FROM Users WHERE Name='" + userInput + "'";
Secure Alternative: Harnessing the Power of Parameterized Queries
To erect a formidable barricade against the perils of inadequate input validation, the judicious adoption of parameterized queries emerges as a sentinel practice. By wielding this technique in the arena of database interactions, developers erect an impregnable defense, preventing malevolent data from surreptitiously metamorphosing into executable code.
string userInput = Request.QueryString["input"];
string sqlQuery = "SELECT * FROM Users WHERE Name=@userName";
SqlCommand command = new SqlCommand(sqlQuery, connection);
command.Parameters.AddWithValue("@userName", userInput);
Memory Handling
Unsafe: Navigating the Quagmire of Array.Copy()
Treading the precarious path of Array.Copy()
devoid of vigilant bounds checking manifests as a perilous gamble, ushering in the specter of calamitous buffer overflows and other labyrinthine memory-related conundrums.
int[] sourceArray = { 1, 2, 3, 4, 5 };
int[] destinationArray = new int[3];
Array.Copy(sourceArray, destinationArray, 5); // Buffer overflow potential
Secure Alternative: Embracing the Haven of Array.CopyTo()
In pursuit of memory handling that evades the clutches of vulnerability, developers can seamlessly transition to the sheltered embrace of Array.CopyTo()
. This conscientious choice empowers developers to orchestrate the secure migration of memory, effectively snuffing out the lurking threat of buffer overflow vulnerabilities.
int[] sourceArray = { 1, 2, 3, 4, 5 };
int[] destinationArray = new int[3];
sourceArray.CopyTo(destinationArray, 0);
File Handling
Unsafe: Navigating the Perilous Waters of File.Delete()
The allure of File.Delete()
harbors a double-edged sword, capable of recklessly decimating files sans proper permissions, and in some harrowing instances, unwittingly triggering the execution of arbitrary files, an unforgiving breach of security’s delicate veil.
string filePath = "path/to/file.txt";
File.Delete(filePath); // Deletes the file without proper permissions
**Secure Alternative: Sentinel of SecureString
for File Paths
To erect an impervious bulwark against the capricious whims of unauthorized file deletion and the lurking specter of security breaches, developers must invoke the vanguard of SecureString. By adorning file paths with this stalwart guardian and entwining it with judicious file access control measures, the developer’s arsenal becomes fortified, repelling potential threats with steadfast resolve.
using System.Security;
// ...
SecureString secureFilePath = new SecureString();
foreach (char c in "path/to/file.txt")
{
secureFilePath.AppendChar(c);
}
// Implement proper file access control using secureFilePath
Cryptography
Unsafe: Forging a Path of Peril with Custom Cryptographic Algorithms
The siren call of crafting bespoke cryptographic algorithms, although tempting, leads down a treacherous trail fraught with perilous security flaws. The absence of rigorous testing and validation morphs these creations into ticking time bombs.
// This is an example of an unsafe custom cryptographic algorithm, DO NOT use this in production
public static string UnsafeCustomEncrypt(string data)
{
// ... custom encryption logic ...
return encryptedData;
}
Secure Alternative: A Beacon of Assurance with Established Cryptographic Libraries
Wise is the developer who anchors their cryptographic pursuits to the bedrock of established and rigorously-vetted cryptographic libraries, revering the tenets of industry standards. Noteworthy exemplars include the esteemed Bouncy Castle library, a stalwart guardian for C# enthusiasts.
using Org.BouncyCastle.Crypto;
using Org.BouncyCastle.Security;
// ...
public static byte[] SecureEncryption(byte[] data)
{
IBufferedCipher cipher = CipherUtilities.GetCipher("AES/ECB/PKCS7Padding");
// ... setup encryption parameters and key ...
return cipher.DoFinal(data);
}
Conclusion: Safeguarding Code for a Secure Tomorrow
In the realm of software development, a singular truth resonates: prioritizing security stands as an imperious imperative. The sentinel guardianship of software integrity unfurls its banner in myriad ways, none more imperative than navigating the labyrinthine alleys of unsafe C# functions. By wielding a discerning gaze that unearths the lurking perils poised by these functions, and concurrently, by ardently embracing the mantle of their secure alternatives, developers metamorphose into architects of fortitude, erecting bastions that defy adversarial exploits and proactively cultivating a terrain fortified against vulnerabilities.
Let the indelible lesson resound: the voyage towards secure software is an unceasing odyssey, a pilgrimage unmarred by its perpetual pursuit. Staying attuned to the symphony of best practices and the mosaic of latent vulnerabilities is the compass that steers developers through the tempestuous seas of uncertainty. As each keystroke shapes the digital universe, a legacy of resilient code emerges, a testament to the unwavering resolve to safeguard the digital realm.
Remember, the road to secure software is a continuous journey, and staying informed about best practices and potential vulnerabilities is key to success.
References
- Microsoft C# Documentation
- OWASP Top Ten
- C# Secure Coding Guidelines
- Bouncy Castle Cryptography Library