Effortless Docker Image Optimization: 8 Proven Tips for Efficiency
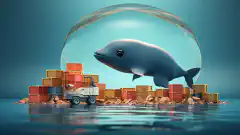
Table of Contents
Optimizing Docker Images for Efficiency, Performance, and Security
In the realm of containerization, Docker reigns supreme. Docker images serve as the foundation of containerized applications, encapsulating every element required for seamless operation, from code to essential libraries and dependencies. However, as applications expand and evolve, Docker images can bloat, resulting in inefficient and resource-intensive containers. In this comprehensive guide, we delve into expert techniques to meticulously optimize Docker images, enhancing efficiency, boosting performance, and fortifying security.
Introduction
Docker images play a pivotal role in the containerization process, but their sheer size can significantly impact multiple facets of your application’s lifecycle. These ramifications include deployment speed, storage overhead, and the overall security posture. Therefore, the art of optimizing Docker images is of paramount importance, ensuring that your containers operate with the utmost efficiency.
In the world of containerization, Docker has become a household name. Docker images are the building blocks of containerized applications. They encapsulate everything an application needs to run, from code to libraries and dependencies. However, as your application grows and evolves, so do your Docker images, often leading to bloated and inefficient containers. In this article, we’ll explore techniques to optimize Docker images for efficiency, performance, and security.
Explore more about Docker optimization and container security in these informative articles:
Choose a Suitable Base Image for Docker Image Optimization
Docker Image Optimization begins with a crucial decision: selecting the ideal base image. This base image serves as the bedrock upon which your application is constructed, determining the underlying Linux distribution and pre-installed packages. When making this choice, consider the following factors:
Alpine Linux: Alpine stands out as a size-optimized base image, boasting a featherweight size of approximately 3 MB. However, it utilizes the musl C-library, which may introduce compatibility challenges. To explore Alpine images on Docker Hub, visit Alpine Linux on Docker Hub .
Example Dockerfile snippet using Alpine as a base image:
FROM alpine:latest
Ubuntu or Debian: These Linux distributions offer compact base images, typically weighing less than 40 MB. Debian even offers a slim tag, further trimming down the image size. You can find Ubuntu and Debian images on Docker Hub.
- Example Dockerfile snippet using Debian as a base image:
FROM debian:slim
- Example Dockerfile snippet using Debian as a base image:
Language-Specific Images: Language-specific images, such as Python or Node.js, often come with substantial default images. However, they provide slim variants that exclude optional packages, resulting in a significantly smaller footprint. Explore language-specific images on Docker Hub.
- Example Dockerfile snippet using a Python slim image:
FROM python:slim
Striking the right balance between image size and compatibility is paramount. Your choice of a base image should align with the specific requirements of your application.
Multi-Stage Builds for Docker Image Optimization
Docker Image Optimization reaches new heights with the utilization of multi-stage builds, an ingenious technique for drastically reducing image size. This strategy involves breaking down the image creation process into distinct stages, typically comprising a “build image” and a “run image.” Let’s delve into the magic of multi-stage builds:
Build Image: The first stage, known as the build image, serves as a workspace equipped with all the essential tools and dependencies required for assembling your application. It’s the space where you can install package managers, compile native code, and execute any other tasks necessary for building your application. This stage focuses on the development and construction aspects of your Docker image.
- Example Dockerfile snippet illustrating a build image:
# Build image stage FROM golang:1.16 AS builder WORKDIR /app COPY . . RUN go build -o myapp # Run image stage FROM alpine:latest WORKDIR /app COPY --from=builder /app/myapp . CMD ["./myapp"]
- Example Dockerfile snippet illustrating a build image:
Run Image: The second stage, referred to as the run image, is the streamlined version of your image, containing only the critical runtime components needed for running your application. Here, you perform a selective copy of the compiled code and essential libraries from the build image, leaving behind any unnecessary build artifacts. This stage is focused on runtime efficiency and results in a significantly smaller final image. Multi-stage builds offer a brilliant solution to the Docker image size dilemma, allowing you to optimize both development and production aspects.
Streamline Docker Image Size with Consolidated RUN Commands
Docker Image Optimization takes a significant leap forward by simplifying the Dockerfile with consolidated RUN
commands. It’s a common practice to use multiple RUN
commands in a Dockerfile to execute various tasks. However, it’s essential to understand that every RUN
command generates a new layer within the image, potentially leading to a bloated image size. Let’s explore the art of consolidating RUN
commands to achieve image size efficiency:
Rather than scattering commands like this throughout your Dockerfile:
RUN git clone https://some.project.git
RUN cd project
RUN make
RUN mv ./binary /usr/bin/
RUN cd .. && rm -rf project
Consider a streamlined approach that not only simplifies your Dockerfile but also optimizes the image size. This approach involves consolidating related tasks into a single RUN
command. By doing so, you reduce the number of image layers and eliminate any unnecessary intermediate files.
Example of a consolidated RUN
command:
RUN git clone https://some.project.git \
&& cd project \
&& make \
&& mv ./binary /usr/bin/ \
&& cd .. && rm -rf project
This optimization technique ensures that temporary files, such as source code, are not retained in the final Docker image, contributing to a more efficient and streamlined container.
Enhance Docker Image Efficiency with Squash Image Layers
When it comes to Docker Image Optimization, the docker-squash
tool emerges as a powerful ally. This Python-based utility offers a seamless way to drastically reduce Docker image size. The magic happens by compressing the last N layers of an image into a single layer, effectively eliminating any redundant files or folders that might have been created and then deleted in previous layers. Squashing, as a technique, shines, especially when dealing with legacy images.
Here’s how you can leverage the docker-squash
tool to enhance Docker image efficiency:
Identify Inefficient Layers: Before squashing, it’s essential to pinpoint which layers of your Docker image need optimization. You can achieve this by using the
docker history <imagename>
command or employing handy tools likedive
that analyze layer efficiency.Streamline and Shrink: Once you’ve identified the culprits, the
docker-squash
tool comes into play. It consolidates those layers, removing any unnecessary baggage and streamlining your Docker image.
Example Docker Image Squashing Command:
docker-squash -t <tagname> <imagename>
By utilizing this approach, you effectively eliminate wasted space in your Docker images, paving the way for more efficient and streamlined containers.
Optimize Docker Image Size by Efficient Dependency Management
In the realm of Docker Image Optimization, handling dependencies plays a pivotal role. Docker image creation often involves the use of package managers such as apt
, yum
, pip
, or npm
to procure essential software components. However, these steps can inadvertently lead to bloated images if not managed judiciously. Here are savvy approaches to save space when installing dependencies for common programming languages:
For Python Projects:
Selective Dependency Installation: When working with Python, you can optimize Docker image size by instructing
pip
to install only the bare essentials, without caching. This helps avoid unnecessary bloat in your Docker image.For instance, you can use the
--no-cache-dir
flag withpip
during installation:pip install --no-cache-dir <package>
For Node.js Projects:
Minimize Unnecessary Dependencies: In Node.js projects, carefully examine your
package.json
file and prune any dependencies that are not essential for your application’s runtime. This step reduces the number of packages installed and, consequently, the image size.You can use the
npm prune --production
command to achieve this:npm prune --production
Cache Cleaning: Similar to other package managers, npm maintains a cache. Cleaning this cache periodically can help free up space in your Docker image. Use the following command:
npm cache clean --force
General Optimization Techniques:
Selective Dependency Installation: One potent technique is to instruct the package manager to install only the bare essentials, excluding recommended packages. This helps avoid unnecessary bloat in your Docker image.
For instance, when using
apt
, you can employ the--no-install-recommends
flag during installation. And fordnf
you can employ--setopt=install_weak_deps=False
.- Debian and Ubuntu Systems using APT
In the case of
apt
, use the following flag during installation:
apt-get install --no-install-recommends <package>
- RHEL, CENTOS, ROCKY, and FEDORA Systems using DNF
In the case of
dnf
, use the following flag during install to achieve the same effect.
dnf -y install --setopt=install_weak_deps=False <package>
- Debian and Ubuntu Systems using APT
In the case of
Cache Cleaning: Another space-saving maneuver involves cleaning the package manager’s cache to prevent superfluous storage consumption. By purging cached data, you trim down the image size.
- Debian and Ubuntu Systems using APT
In the case of
apt
, use the following command:apt-get clean -y
- RHEL, CENTOS, ROCKY, and FEDORA Systems using DNF
In the case of
dnf
, use the following command to achieve the same effect.dnf clean all
- Debian and Ubuntu Systems using APT
In the case of
These optimizations are indispensable for crafting lean and efficient Docker images. However, it’s crucial to note that each package manager and programming language may have its specific commands and options for implementing these strategies. To ensure you’re making the most of these techniques, consult the documentation tailored to your chosen package manager and programming language.
Docker Image Optimization: Avoid Superfluous chowns
In the realm of Docker Image Optimization, it’s crucial to avoid superfluous chown
operations. Modifying files in the build container, including changing ownership or permissions, can result in image bloat. Docker creates a new copy of a file whenever it is modified in any way, including changes in ownership or permissions. To minimize duplication and optimize your Docker image size, use the --chown
option with the COPY
command to perform ownership changes in a single step, ensuring only one instance of the files is created.
Here’s an example of how to use the --chown
option in your Dockerfile:
COPY --chown=youruser code .
instead of:
COPY code .
RUN chown -R youruser code
This approach is more efficient and avoids unnecessary file duplication, ultimately reducing your Docker image size.
For more details on optimizing Docker images and using the --chown
option, you can refer to the
Docker documentation
.
Avoiding superfluous chowns
is a fundamental aspect of Docker Image Optimization, contributing to smaller, more efficient Docker containers.
Docker Image Optimization: Use .dockerignore Files
One of the essential strategies for Docker Image Optimization is using .dockerignore
files. These files allow you to specify files and folders that should be excluded from the Docker image during the build process. By using .dockerignore
, you prevent large or unnecessary files from being copied into the image, reducing its size and build time.
For example, you can exclude test data files, development artifacts, or logs that are not required for the production runtime environment. This not only optimizes image size but also ensures that your Docker image contains only essential components.
To create a .dockerignore
file, you can use a simple text editor like Notepad on Windows or nano on Linux. Here’s an example of a .dockerignore
file for a Node.js application:
# Ignore development-related files and folders
node_modules/
npm-debug.log
Dockerfile.dev
.git/
.gitignore
# Ignore test data
test/
This file instructs Docker to exclude the listed files and directories during image creation. It’s a powerful tool for keeping your Docker images lean and efficient.
For more information on how to create and use .dockerignore
files, you can refer to the
official Docker documentation
.
Incorporating Docker Image Optimization techniques, such as using .dockerignore
files, is crucial for creating efficient and streamlined Docker containers.
Docker Image Optimization: Use the docker-slim Tool
In the world of Docker Image Optimization, the docker-slim
tool emerges as a potent solution. This tool operates by analyzing your application’s behavior within a temporary container, pinpointing the files and folders genuinely utilized during runtime. Subsequently, docker-slim
crafts a new single-layer image, incorporating only these crucial components.
Notable advantages of docker-slim
encompass:
- Extremely Compact Images: Docker images produced by
docker-slim
are often smaller than those based on Alpine, resulting in leaner containers. - Enhanced Security: Unnecessary tools and libraries are stripped away by
docker-slim
unless explicitly mandated by your application, bolstering image security. - Simplified Dockerfile Management:
docker-slim
reduces reliance on other optimization techniques, streamlining Dockerfile management.
It’s imperative to exercise caution when using docker-slim
. Thorough testing and configuration are essential. Misconfigurations may inadvertently remove files required for specific edge cases. To mitigate this risk, maintain explicit “preserve path” lists and utilize dynamic probes to ensure your application’s dependencies are accurately identified.
For detailed instructions on implementing docker-slim
and optimizing your Docker images, refer to the
docker-slim documentation
.
Conclusion: Mastering Docker Image Optimization
In the realm of containerization, achieving peak performance and resource efficiency is paramount. Docker images play a pivotal role in this endeavor. By embracing the principles of Docker Image Optimization, you can significantly enhance container efficiency, cut down operational costs, and fortify the security of your containerized applications.
Here’s a recap of the key strategies covered in this guide:
Select the Right Base Image: Begin your optimization journey by choosing a suitable base image. Options like Alpine Linux, Ubuntu, and Debian offer various trade-offs between size and compatibility. Tailor your choice to your application’s specific needs.
Leverage Multi-Stage Builds: Divide the image creation process into distinct stages—build and run. This segregation empowers you to craft lean images by eliminating unnecessary build artifacts, ultimately leading to a smaller image footprint.
Consolidate RUN Commands: Streamline your Dockerfile by merging related
RUN
commands. This reduces the number of image layers, resulting in a more compact image and improved efficiency.Squash Image Layers: Use tools like
docker-squash
to merge multiple image layers into one. This eliminates redundant files and optimizes legacy images, reducing overall image size.Save Space When Installing Dependencies: When installing dependencies, instruct the package manager to include only essential components and perform cache cleaning to prevent unnecessary storage consumption. Consult your package manager’s documentation for precise commands.
Avoid Superfluous
chown
Operations: Minimize image bloat by employing the--chown
option with theCOPY
command. This ensures ownership changes occur in a single step, reducing file duplication and enhancing image efficiency.Use
.dockerignore
Files: Craft a.dockerignore
file to specify which files and directories to exclude from your Docker image. This practice minimizes image size and build time by excluding non-essential components.Leverage the
docker-slim
Tool: Embrace the power ofdocker-slim
to create ultra-compact Docker images. This tool analyzes your application’s behavior, leading to extremely small images, bolstered security, and simplified Dockerfile management.
In conclusion, it’s crucial to understand that Docker image optimization isn’t a one-size-fits-all endeavor. The techniques you employ should align with your application’s unique demands and constraints. However, by implementing these best practices and staying attuned to the evolving Docker landscape, you can ensure your Docker images are finely tuned for efficient containerization.
Embrace Docker Image Optimization to master the art of containerization and propel your applications toward peak performance and security.
References
This article refers to other articles we've written:
- Building Efficient and Secure Docker Containers: A Guide for Beginners
Learn how to create efficient and secure Docker containers using best practices, tips, and step-by-step instructions in this comprehensive guide.
- How to Secure Your Docker and Kubernetes Environment
Learn best practices for securing your Docker and Kubernetes environment, including using official images, limiting permissions, and implementing network security.